728x90
반응형
프렌드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
|
#include <iostream>
#include<string>
using namespace std;
// a=(1,2) b=(2,3) 일 때 a+b=(3,5) 라고 하는게 연산자 중복
//프렌드
// 클래스의 멤버가 아닌 외부 함수
// 프렌드 후보 -> 전역함수, 다른 클래스의 멤버함수
// 클래스의 모든 멤버 접근할 수 있는 권한부여
//왜? 다른 외부 함수인데 내 멤버 variable 열고 싶을 때 씀
//멤버는 프렌드가 아님
//프렌드가 여러개여도 ㄱㅊㄱㅊ
class rect; //컴파일 오류를 막기 위함
class rectmanager {
public:
bool equal(rect a, rect b);
};
class rect {
public:
int height;
int width;
rect(int width, int height) {
this->height = height;
this->width = width;
}
/*friend bool equlas(rect r, rect c);*/
friend bool rectmanager::equal(rect a,rect b);
////원래는 private 영역에 있는 애들 못가져오는데 friend 여서 가지고 올 수 있음
/*friend rectmanager*/;
};
bool rectmanager::equal(rect a, rect b) {
if (a.width == b.width && a.height == b.height) {
return true;
}
return false;
}
int main() {
rect a(3, 4);
rect b(3, 4);
rectmanager man;
cout<<man.equal(a,b)<<endl;
//rectmanager의 equal함수에 접근하고 싶으면 어쨌든 equal은
//rectmanager에 있기에 객체 만들어주고
}
|
cs |
연산자 오버로딩
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
#include <iostream>
#include<string>
using namespace std;
//+ 기호를 다른 곳에서도 써보자! -> 연산자 오버로딩
// 하는 이유는 보기 편해서!
//Red.plus(Green) 보다 Red+Green이 더 직관적
class Color {
string s;
public:
Color(string s) {
this->s = s;
}
//프렌드로 선언하는 경우
// 매개변수가 두개 필요함
//+(a,b)
friend Color operator+(Color c1, Color c2);
string getString() {
return s;
}
// 클래스의 멤버함수로 선언하는 경우
// 매개변수가 한개 필요함
//a.+(b)
//Color operator+(Color c1);
};
Color operator+(Color c1, Color c2);
int main() {
// 더하기에 대한 새로운 정의
//연산자 오버로딩
// 1.클래스의 멤버함수로 구현
// 2.외부에서 구현한다음에 클래스에 프렌드함수로 선언 -> 클래스의 멤버 변수에 접근해야해서
// 형태: 리턴타입 operator연산자(매개변수 리스트)
Color a("Blue");
Color b("Red");
Color c = a + b;
}
|
cs |
연산자 오버로딩2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
|
#include <iostream>
#include <string>
using namespace std;
class Power {
int punch;
int kick;
public:
Power(int kick = 0, int punch = 0) {
this->kick = kick;
this->punch = punch;
}
// + 연산자
Power operator+(Power op2);
//== 연산자
bool operator==(Power op2);
//+=연산자
Power& operator+=(Power op2);
//단항연산자 ++ -- (피연산자가 하나뿐인 연산자)
//전위 증가
Power& operator++();
//후위 증가
Power operator++(int x);
bool operator!();
//프렌드를 써보자
friend Power operator+(int op1, Power op2);
void show();
};
Power Power::operator+(Power op2) {
// c=a.+(b) 와 같음. 어차피 a는 없음
Power tmp;
tmp.kick = this->kick + op2.kick;
tmp.punch = this->punch + op2.punch;
return tmp;
}
bool Power::operator==(Power op2) {
if (this->kick == op2.kick && this->punch == op2.punch) {
return true;
}
return false;
}
Power& Power::operator+=(Power op2) {
kick = this->kick + op2.kick;
punch = this->punch + op2.punch;
return *this; //자신의 참조 리턴
}
//전위는 ++a 후위는 a.++()
Power& Power::operator++() {
kick++;
punch++;
return *this;
}
//근데 매개변수 x가 안쓰임
//근데 매개변수 안쓰면 컴파일에러 뜸
//input과 함수명이 같으면 중복이 일어나고 모호성
//int x 가 없으면 얘가 전위인지 후위인지모름
//매개변수가 있으면 후위다! 라는걸 구별시켜줘서 모호성 사라지게 하기 위함
//즉, 함수 호출의 모호성을 없앤다.
Power Power::operator++(int x) {
Power tmp = *this;
kick++;
punch++;
return tmp;
}
bool Power::operator!() {
//kick,power가 모두 0이면 true
if (kick == 0 && punch == 0) {
return true;
}
return false;
}
void Power::show() {
cout << "kick: " << kick << "," << "punch: " << punch << endl;
}
Power operator+(int op1, Power op2) {
Power tmp;
tmp.kick = op2.kick + op1;
tmp.punch = op2.punch + op1;
return tmp;
}
int main() {
// 멤버함수로 이항 연산자 구현
// a+b (항이 2개)
Power a(3, 5);
Power b(4, 6);
Power c;
c = a + b;
Power d;
d += a;
a.show();
b.show();
b = ++a;
b.show();
c.show();
d.show();
if (a == b) {
cout << "두 파워가 같다." << endl;
}
else {
cout << "두 파워가 같지 않다." << endl;
}
Power e(3, 10);
Power k;
k = 3 + e; //3+e 여야 e+3아님!!
e.show();
k.show();
}
|
cs |
연산자 오버로딩에 프렌드를 쓰면?

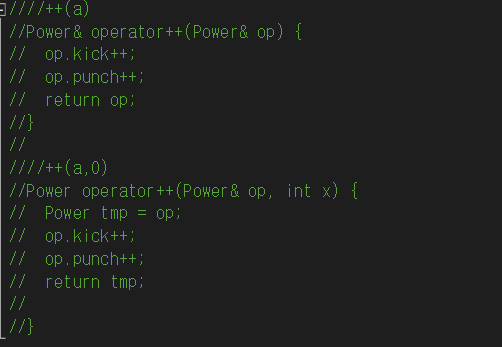
728x90
반응형
'C++' 카테고리의 다른 글
[C++] 순수가상함수와 추상클래스 (0) | 2022.12.04 |
---|---|
[C++] 상속과 접근지정자 정리 (0) | 2022.12.03 |
[명품 c++] 3장 실습문제 (0) | 2022.10.18 |
[C++] 명품 C++ 4장 찝어준거 정리 (0) | 2022.10.14 |
[C++] 명품 c++ 3장 찝어준거 정리 (0) | 2022.10.14 |
댓글